How For loop works in C# with example code snippet
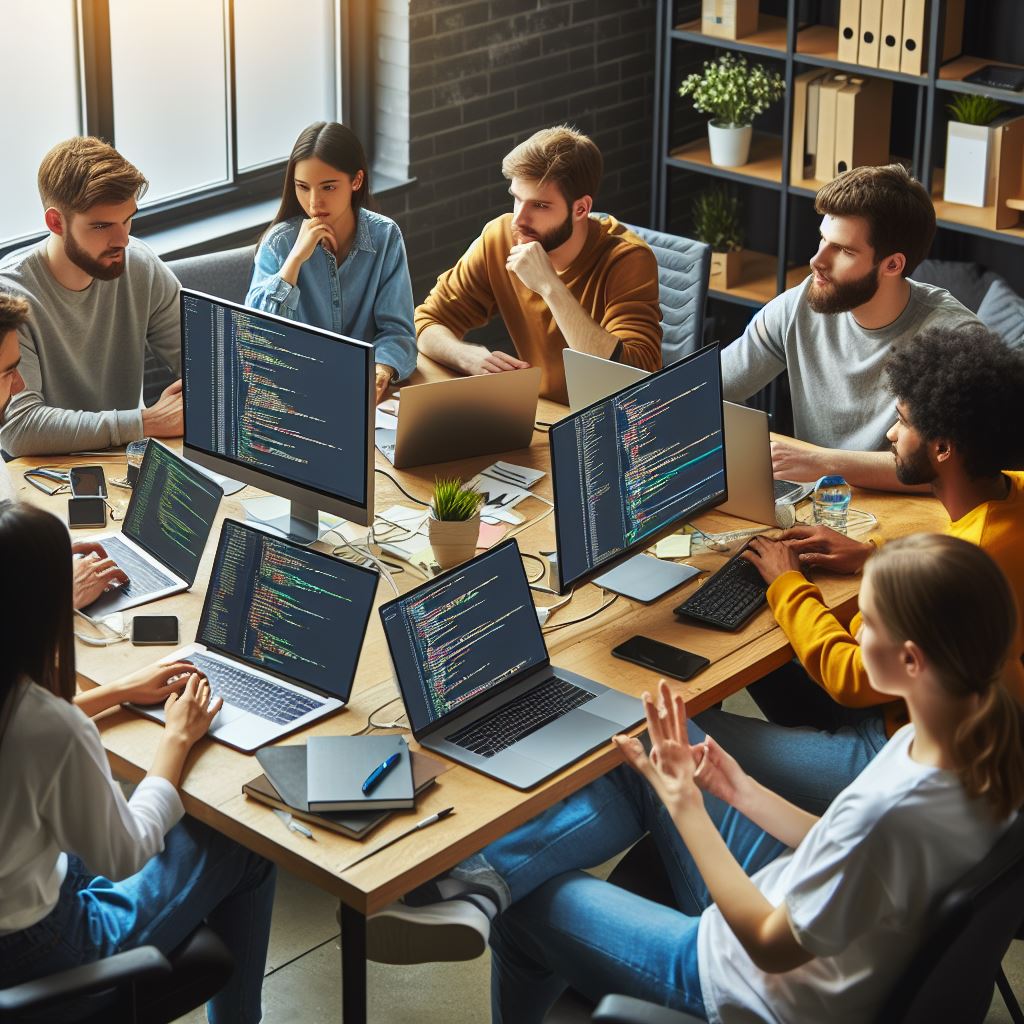
In C#, a for
loop is used to execute a block of code repeatedly based on a condition. It consists of three parts:
- Initialization: Initialize the loop control variable.
- Condition: Check if the loop control variable meets the condition.
- Iteration: Update the loop control variable.
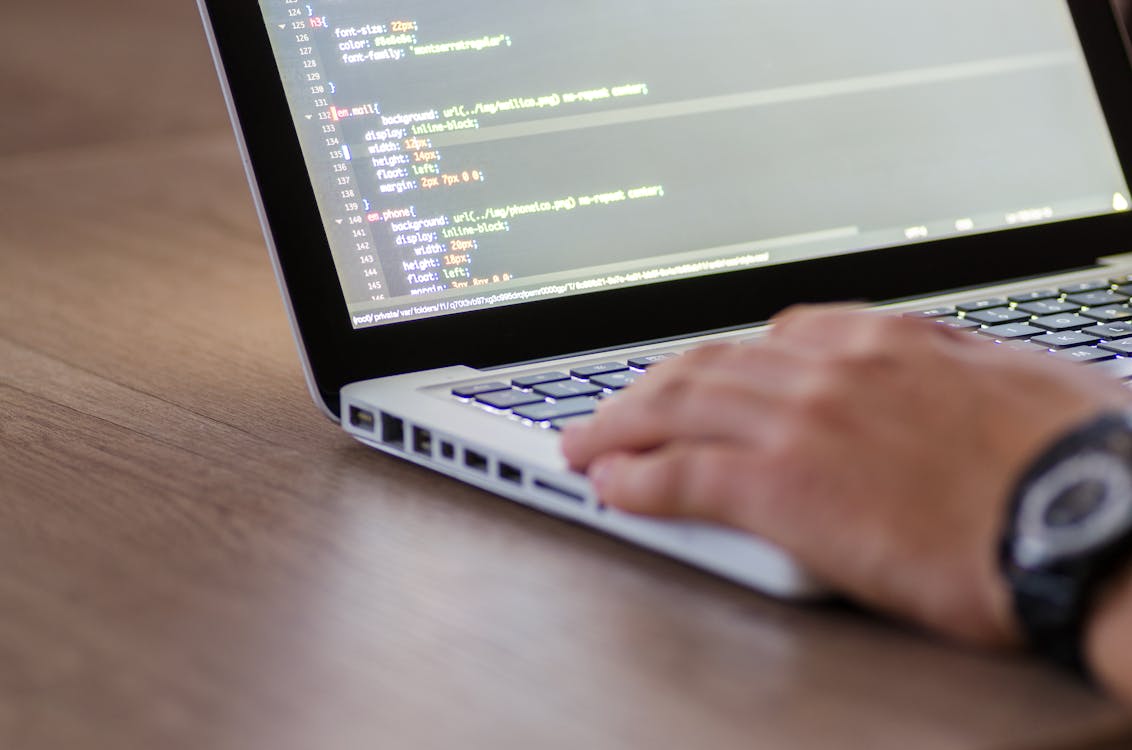
Here’s a simple example of a for
loop in C#:
using System;
class Program
{
static void Main(string[] args)
{
// Example: Printing numbers from 1 to 5 using a for loop
for (int i = 1; i <= 5; i++)
{
Console.WriteLine(i);
}
}
}
Explanation of the for
loop in the example:
int i = 1
: Initialization – Initialize the loop control variablei
to 1.i <= 5
: Condition – Check if the loop control variablei
is less than or equal to 5.i++
: Iteration – Increment the loop control variablei
by 1 in each iteration.
The loop will execute as follows:
i
is initialized to 1.- Check if
i
is less than or equal to 5. Since it’s true, execute the loop body (printingi
) and then incrementi
. - Repeat the process until the condition
i <= 5
becomes false.
The loop will print numbers from 1 to 5, and then terminate.Hope this helps.